자바스크립트 객체
prototype를 사용해서 자바스크립트 클래스를 만들 수 있다. 자바스크립트에는 Date, String 등의 클래스가 있고 이렇게 기본적으로 제공하는 클래스 외에 추가적으로 개발자가 직접 새로운 클래스를 정의할 수 있다.
① 출력 기능의 로그 스크립트 작성 - log.js
![]() function log(msg){ var consolE = document.getElementById("console"); consolE.innerHTML += msg + "<br/>"; } |
② Member클래스 생성
자바스크립트를 이용해서 클래스를 생성할 수 있다.
Member클래스 생성 후 생성자를 가지고 객체를 초기화시킨 것과 동일. 객체 생성시 id와 name 두 파라미터값을 전달받는 클래스를 정의했다. Member = function(id,name,addr){ this.id = id; this.name = name; this.addr = addr; }; 클래스 안에 함수 정의(setter) 멤버안에 메소드를 만들고 메소드로 초기화시킨 것과 동일 클래스가 제공할 함수를 정의할 땐 prototype를 사용한다. Memeber.prototype.setValue = function(id,name,addr){ this.id = id; this.name = name; this.addr = addr; }; 클래스 안에 함수 정의(getter). 리턴하는 함수도 사용가능 Member.prototype.getValue = function(){ return "[" + this.id + "]" +this.name + "(" + this.addr + ")"; }; |
③ JSP 페이지 - 객체 생성 및 출력
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>prototype활용</title> <script type="text/javascript" src="<%=cp%>/data/js/log.js"></script> <script type="text/javascript" src="<%=cp%>/data/js/member.js"></script> <script type="text/javascript"> function memberClass(){ //1.member.js 없이 실행(메모리에 객체 생성) var object1={};//object1이라는 객체를 만든 것. object1.id = "test"; object1.name = "홍길동"; log("log1: "+object1.id +" ," +object1.name ); //2.member.js 없이 실행(메모리에 객체 생성) var object2={};//object2이라는 객체를 만든 것. object2.id = "hoho"; object2.name = "신난다"; log("log2: "+object2.id +" ," +object2.name ); //3.Member클래스 객채 생성 후 변수명으로 호출 var member = new Member("soso","소소한끼","강남");//생성자처럼 사용함 log("member1: "+member.id +" ," +member.name +"," +member.addr); //4.setter로 초기화하고 변수로 호출 member.setValue("HL","ㅎㄹ","제주도"); log("member2: "+member.id +" ," +member.name +"," +member.addr); //5.getter로 호출 var memberInfo = member.getValue(); log("member.getValue(): "+ memberInfo); } window.onload = function(){ memberClass(); } </script> </head> <body> <h1>JavaScript 클래스 사용</h1> <hr/> <div id="console"></div> </body> </html> |
④ 출력 페이지
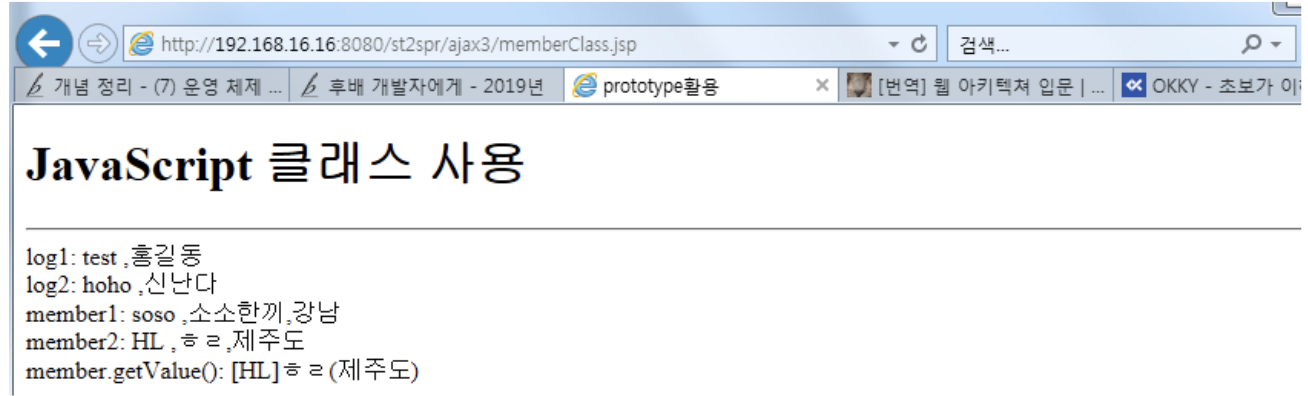
'Dev > JS & Jquery' 카테고리의 다른 글
Ajax - 방명록 만들기 (0) | 2019.04.03 |
---|---|
Ajax - JSON을 활용하여 자바스크립트의 객체 생성 (0) | 2019.04.03 |
Ajax - Document Object Model과 XML (0) | 2019.04.03 |
Ajax - 검색시 제시어 기능 만들기 (0) | 2019.04.02 |
Ajax - get/post방식,Id유효성검사,서버/클라이언트 시간확인,CSV형식 데이터 읽기 (0) | 2019.04.01 |