JSON 표기법
JavaScript Object Notation 표기법은 서로다른 프로그래밍 언어 간에 데이터를 교환하기 위한 표기법으로 읽고 쓰기 쉬운 표기법이다.
DB에 있는 데이터를 JSON표기 형식으로 변환하기 위해 소스 코드를 작성하는 방법도 공부하자!
① Key: Value 쌍
Map이나 hashTable과 같은 방식. key는 String이다.
collection 타입. 다양한 언어들에서, 이는 object, record, struct(구조체), dictionary, hash table, 키가 있는 list, 또는 연상배열로서 구현
{ “이름1”:값1, “이름2”:값2, “이름3”:값3 }
② 배열
값들의 순서화된 Collection. 대부분의 언어들에서 array, vector, list, 또는 sequence로서 구현된다.
들어갈수 있는 값으로는 큰따옴표안에 string, number ,true ,false , null, object ,array이 올수있다
JSON 데이터에 접근할 때는 객체.이름이나 객체[이름]의 두가지 방식중 하나를 사용해서 접근할 수 있다.
배열에 접근할 떄는 객체[인덱스] 형식으로 접근할 수있으며 이때 값은 0 부터 시작한다.
[값0, 값1, 값2, 값3]
③기본적인 형태
(1) 프로퍼티 : 값 var obj = { "프로퍼티 이름" : "값",
(2) 메소드 var obj = { "메소드 이름" : function() {alert('This is method')} }
(3) 메소드(인수) var obj = { "메소드 이름" : function(인수) {alert('This is method')} }
이것만으로 객체 obj를 만드는 것이 가능하다.
obj.프로퍼티이름 으로 값을 얻어 낼 수 있어, obj.메소드이름() 으로 "This is method"라는 대화창을 표시한다.
자바스크립트의 객체 생성
① 자바스크립트 소스 파일 생성 - member_json.js
var com = new Object(); com.util = new Object(); com.util.Member = function(id,name,addr){ this.id = id; this.name = name; this.addr = addr; }; //메소드생성 com.util.Member.prototype={ //setter setValue:function(id,name,addr){ this.id = id; this.name = name; this.addr = addr; }, setId:function(id){ this.id = id; }, setName:function(name){ this.name = name; }, setAddr:function(addr){ this.addr = addr; }, //getter getValue:function(){ return this.id + ":" + this.name +":" + this.addr; }, getId:function(){ return this.id; }, toString:function(){ return this.id + ":" + this.name +":" + this.addr; } } |
② 객체 생성 페이지 - memberJSONClass.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <script type="text/javascript" src="<%=cp%>/data/js/log.js"></script> <script type="text/javascript" src="<%=cp%>/data/js/member_json.js"></script> <script type="text/javascript"> function memberClass(){ //객체 생성 var member = new com.util.Member("aaa","홍길동","서울"); log("member1 : " + member.id +"," + member.name + "," + member.addr); //setter 이용 member.setValue("bbb","가나다","부산"); log("member2 : " + member.id +"," + member.name + "," + member.addr); //getter 이용 var memberInfo = member.getValue(); log("member.getValue : " + memberInfo); member.setId("hahahoho"); log("member.toString() : " + member.toString()); var id = member.getId(); log("member.getId() : " + id); } window.onload = function(){ memberClass(); } </script> </head> <h1>JavaScript 클래스 사용 - JSON</h1> <hr/> <div id="console"></div> </body> </html> |
③ 실행 화면
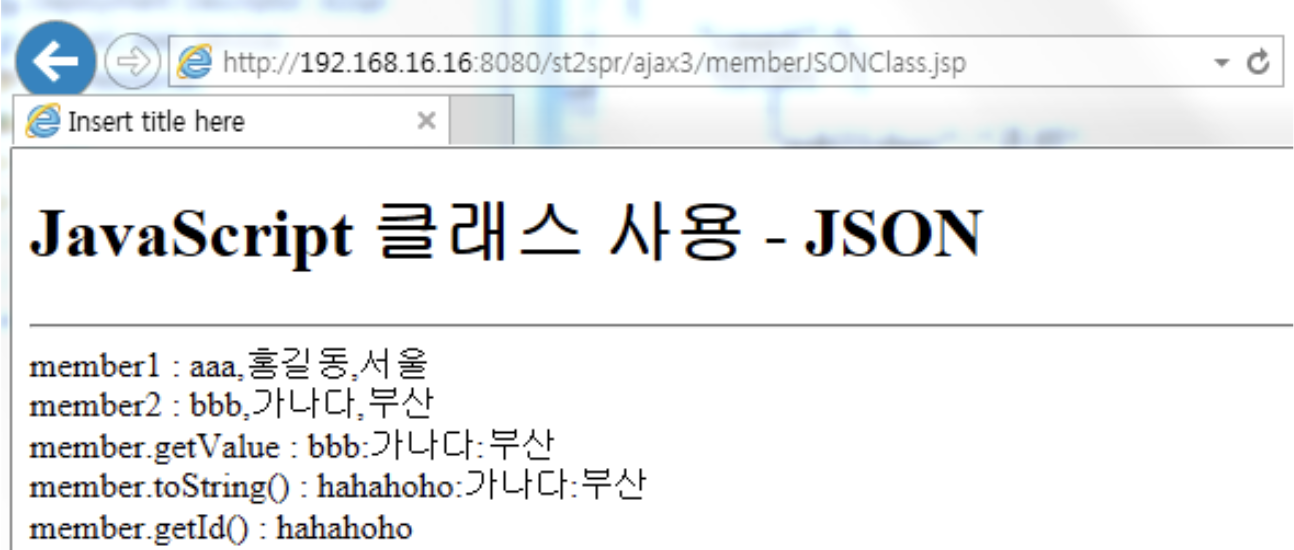
JSON 데이터 읽기
① JSON 데이터 읽어오기 - newsTitleJSON.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>newsJSON</title> <style type="text/css"> .news{ font-size: 9pt; display:block; margi:0 auto; background: yellow; color: blue; border: 1px dashed black; width: 50%; } .newserror{ font-size: 9pt; display:block; margi:0 auto; background: red; color: red; border: 1px dashed black; width: 50%; } </style> <script type="text/javascript" src="<%=cp%>/data/js/httpRequest.js"></script> <script type="text/javascript"> function newsTitle(){ sendRequest("newsTitleJSON_ok.jsp", null, displayNewsTitle, "GET"); //setTimeout("newsTitle()", 3000);//3초마다 자신 호출 } function displayNewsTitle(){ if(httpRequest.readyState==4){ if(httpRequest.status==200){ var jsonStr = httpRequest.responseText; //alert(jsonStr); //eval() 내장함수 //변수를 javaScript의 함수처럼 사용하는 명령어 var jsonObject = window.eval('('+jsonStr+')'); //alert(jsonObject);//Object가 반환됨 //alert(jsonObject.count);//5 //alert(jsonObject.titles[2].publisher); //alert(jsonObject.titles[2].headline); var count = jsonObject.count; if(count>0){ var htmlData = "<ol>"; for(var i=0;i<jsonObject.titles.length;i++){ var publisherStr = jsonObject.titles[i].publisher; var headlineStr = jsonObject.titles[i].headline; htmlData +="<li>" + headlineStr + " [" + publisherStr + "]</li>"; } htmlData +="</ol>"; var newsDiv = document.getElementById("newsDiv"); newsDiv.innerHTML = htmlData; } } } } window.onload = function(){ newsTitle(); }; </script> </head> <body> <h1>NewsTitleJSON</h1> <h2>헤드라인 뉴스</h2> <hr> <br> <div style="width:50%; margin:0 auto;" >뉴스보기</div> <div id="newsDiv" class="news"></div> </body> </html> |
② JSON 데이터 반환 - newsTitleJSON_ok.jsp
<%@ page contentType="text/plain; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> { "count":5, "titles":[ { "publisher":"조선", "headline":"강화된 외감... 대기업마저 '회계포비아'" }, { "publisher":"한국", "headline":"반도체株 바닥 다지고 상승할까 ..." }, { "publisher":"한겨레", "headline":"알아두면 유용한 보험계약 관리 노하우" }, { "publisher":"동아", "headline":"[신율의 정치 읽기] '민족주의' '이념대립' ..." }, { "publisher":"중앙", "headline":"[나기자의 Activity] 일렉트로복싱 해보니" } ] } |
③ 실행 화면
JSON 데이터 읽기
① JSON 데이터 읽어오기 - newsTitleJSON1.jsp
<script type="text/javascript"> function newsTitle(){ sendRequest("newsTitleJSON1_ok.jsp", null, displayNewsTitle, "GET"); //setTimeout("newsTitle()", 3000);//3초마다 자신 호출 } </script> |
② 텍스트 배열 데이터를 JSON 형식으로 변환하여 반환 - newsTitleJSON1_ok.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <%! String[] newsTitle = { "ㅎㅎ 2000호 특별기획 '핀테크' 성지 룩셈부르크를 가다 ...", "강화된 외감... 대기업마저 '회계포비아'", "반도체株 바닥 다지고 상승할까 ...", "알아두면 유용한 보험계약 관리 노하우", "[신율의 정치 읽기] '민족주의' '이념대립' ...", "[나기자의 Activity] 일렉트로복싱 해보니", "[카드뉴스] 'SUV 게 섰거라' 세단의 반격" }; String[] newsPublisher={"중앙","한국","동아","한겨레","조선","CNN","디스패치"}; %> { "count":<%=newsTitle.length %>, "titles":[ <% for(int i=0;i<newsTitle.length;i++){ out.print("{"); out.print("headline:\"" + newsTitle[i]+"\""); out.print(","); out.print("publisher:\"" + newsPublisher[i]+"\""); out.print("}"); if(i!=(newsTitle.length-1)){ out.print(","); } } %> ] } |
③ 실행 화면
'Dev > JS & Jquery' 카테고리의 다른 글
[javascript] 콜백지옥을 탈출하는 q.js (0) | 2020.02.18 |
---|---|
Ajax - 방명록 만들기 (0) | 2019.04.03 |
Ajax - 자바스크립트 클래스 만들기 (0) | 2019.04.03 |
Ajax - Document Object Model과 XML (0) | 2019.04.03 |
Ajax - 검색시 제시어 기능 만들기 (0) | 2019.04.02 |