1. Javascript를 통한 ajax 구현
① helloAjax.jsp - 요청 전송 페이지
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <script type="text/javascript" src="<%=cp%>/data/js/ajaxUtil.js"></script> <script type="text/javascript"> var XMLHttpRequest; function ajaxRequest(){ XMLHttpRequest = createXMLHttpRequest();//객체 생성 XMLHttpRequest.open("GET","helloAjax_ok.jsp",true); //요청을 초기화하면서 요청 방식, 주소, 동기화 여부를 지정. XMLHttpRequest.onreadystatechange = viewMessage; //Callback함수 XMLHttpRequest.send(null);//요청을 보낸다. } function viewMessage(){ var responseText = XMLHttpRequest.responseText;//helloAjax_ok.jsp를 갔다가 넘어오는 response는 Text var divE = document.getElementById("printDIV"); divE.innerHTML = responseText; } </script> </head> <body> <h1>Hello Ajax</h1> <input type="button" value="클릭" onclick="ajaxRequest();"/> <br/> <br/> <div id="printDIV" style="border: 1px solid red; width:50%;"> </div> <br/> </body> </html> |
② helloAjax.jsp - 응답 페이지
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); //서버에서 연산 작업이 이루어지는지 확인하고자 콘솔창에 출력함 for(int i=1;i<300;i++){ System.out.print("delay..."); } %> 오늘은 월요일 어려운 Ajax! |
③ 실행 과정 및 결과
콘솔 실행 화면

(1) 사용자가 웹 브라우저 주소 칸에 "http://localhost:8080/st2spr/ajax2/hello.ajax" 입력
▶ 웹 브라우저는 웹 서버로 http 요청 전송. (2) 웹 서버는 요청한 JSP 페이지를 웹 브라우저로 돌려준다.
(3) 사용자가 버튼을 클릭하면 이벤트가 발생해서 ajaxRequest() 메서드가 실행된다.
(4) XMLHttpRequest 객체가 생성되고 viewMessage() 콜백 함수가 XMLHttpRequest 객체의 onreadystatechange 속성에 저장된다.
(5) GET/비동기 방식으로 서버에 요청을 보내는데, 이때 XMLHttpRequest 객체는 서버의 helloAjax_ok.jsp 파일을 요구한다.
(6) 서버는 Ajax 클라이언트의 요청 URL 인 helloAjax_ok.jsp 을 찾아서 읽은 후에 string 형식으로 XMLHttpRequest 객체로 보낸다.
(7) 콜백 메서드는 XMLHttpRequest 의 state 가 변할 때 실행되므로 readyState=4 이고 stat=200일 때 결과 값을 브라우저에 보낸다.
2. Ajax - Get방식과 Post방식 비교
① ajaxGetPost.jsp - 요청 전송 페이지
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>AjaxGetPost</title> <script type="text/javascript" src="<%=cp%>/data/js/ajaxUtil.js"></script> <script type="text/javascript"> var XMLHttpRequest; function ajaxRequestGet(){ XMLHttpRequest = createXMLHttpRequest();//객체생성 var data = document.myForm.greeting.value;//Form의 데이터 가져오기 if(data==""){ alert("데이터를 입력하세요!"); document.myForm.greeting.focus(); return; } //Get방식(한글깨짐) XMLHttpRequest.open("GET","ajaxGetPost_ok.jsp?greeting="+data,true);//요청 초기화 XMLHttpRequest.onreadystatechange = viewMessage;//callback함수 XMLHttpRequest.send(null);//전송 } function ajaxRequestPost(){ XMLHttpRequest = createXMLHttpRequest();//객체생성 var data = document.myForm.greeting.value; if(data==""){ alert("데이터를 입력하세요!"); document.myForm.greeting.focus(); return; } //POST방식(한글도 잘 전송됨) XMLHttpRequest.open("POST","ajaxGetPost_ok.jsp",true);//요청 초기화 XMLHttpRequest.setRequestHeader("Content-type","application/x-www-form-urlencoded"); XMLHttpRequest.onreadystatechange = viewMessage;//callback함수 XMLHttpRequest.send("greeting="+data);//POST방식은 send할때 파라미터 전송 } function viewMessage(){ var divE = document.getElementById("printDIV"); //1:요청페이지 정보설정, 2:서버에 요청을 보내기 시작, 3:서버에서 요청처리 if(XMLHttpRequest.readyState==1||XMLHttpRequest.readyState==2|| XMLHttpRequest.readyState==3){ //서버의 처리완료시 4가 반환됨. divE.innerHTML = "<image src='../image/processing.gif' width='50' height='50' />";//이미지태그 출력 }else if(XMLHttpRequest.readyState==4){ divE.innerHTML = XMLHttpRequest.responseText;//response로 넘어오는 text 할당 }else{ divE.innerHTML = "<font color='red'>"+XMLHttpRequest.status +" 에러가 발생했습니다."+ "</font>"; } } </script> </head> <body> <h1>AjaxGetPost</h1> <hr/> <form action="" name="myForm"> <input type="text" name="greeting"/> <input type="button" value="Get전송" onclick="ajaxRequestGet();"/> <input type="button" value="Post전송" onclick="ajaxRequestPost();"/> </form> <br/><br/> <div id="printDIV" style="border: 1px solid blue; width:50%"> </div> </body> </html> |
② 사용할 Gif 애니메이션를 원하는 경로에 저장
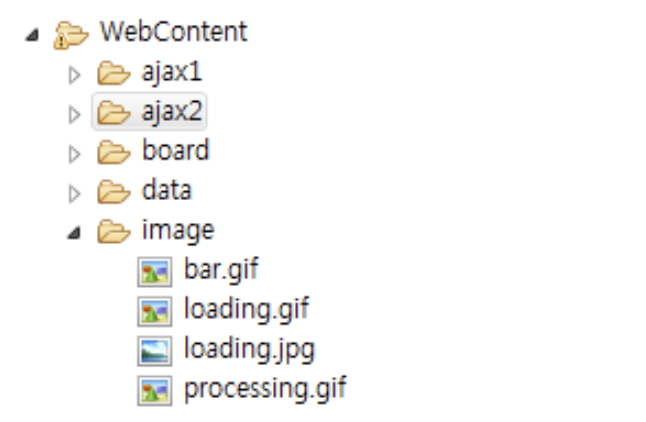
③ ajaxGetPost_ok.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); //프로토콜의 버전 확인 String protocol = request.getProtocol(); System.out.print(protocol); //클라이언트 캐쉬 제거 response.setHeader("Pragma", "no-cache"); response.setHeader("Cache-Control", "no-store");//HTTP 1.0버전 response.setHeader("Cache-Control", "no-cache");//HTTP 1.1버전 프로토콜의 버전을 확인해서 클라이언트의 캐시 제거. 위의 소스와 동일함 //세션 삭제 후(로그아웃) 뒤로가기 방지 response.setDateHeader("Expires", 0); %> <% String greeting = request.getParameter("greeting"); for(int i=0;i<35000;i++){ System.out.println("처리중입니다.."); } %> <%="Server: " + greeting %> |
③ 실행 결과
Get방식을 통한 데이터 전송(전송 완료시 까지 이미지태그가 실행됨)

Post방식을 통한 데이터 전송(인코딩하여 전송하므로 한글도 잘 전송됨)
콘솔 실행 화면

3. Ajax를 활용한 유효한 ID 체크하기
① AjaxID.jsp - 요청 전송 페이지
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <script type="text/javascript" src="<%=cp%>/data/js/ajaxUtil.js"></script> <script type="text/javascript"> var XMLHttpRequest = createXMLHttpRequest(); //객체생성 function requestGet(){ f = document.myForm; if(!f.userId.value){ alert("아이디를 입력하세요!"); f.userId.focus(); return; } var params = "?userId="+f.userId.value; XMLHttpRequest.open("GET","ajaxID_ok.jsp"+params,true); XMLHttpRequest.onreadystatechange = viewMessage; XMLHttpRequest.send(null); } function viewMessage(){ if(XMLHttpRequest.readyState==4){ if(XMLHttpRequest.status==200){ //서버 응답 이상 없음 var str = XMLHttpRequest.responseText; var divE = document.getElementById("resultDIV"); divE.innerHTML = str; } }else{ var divE = document.getElementById("resultDIV"); divE.innerHTML = "<img width='15' height='15' src='../image/loading.gif'/>"; } } </script> <title>AjaxID</title> </head> <body> <h1>Ajax ID 확인</h1> <hr> <form action="" name="myForm"> 아이디 : <input type="text" name="userId" onkeyup="requestGet();"/> </form> <div id="resultDIV" style="color:red; border: 1px solid #000000; width: 40%"> </div> </body> </html> |
② AjaxID_ok.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); String userId = request.getParameter("userId"); String str = ""; if(!userId.equals("")){ for(int i=0;i<30000;i++){ System.out.println("delay..."); } //DB에 쿼리를 이용해서 읽어왔다고 가정하에 진행 if(userId.startsWith("angelina")){ str = userId +"는 유효한 아이디 입니다."; }else{ str = userId +"는 유효한 아이디가 아닙니다."; } } %> <%=str%> |
실행 화면
onkeyup의 이벤트를 사용하여 키보드를 누르고 뗄 때마다 requestGet()메소드가 실행된다.

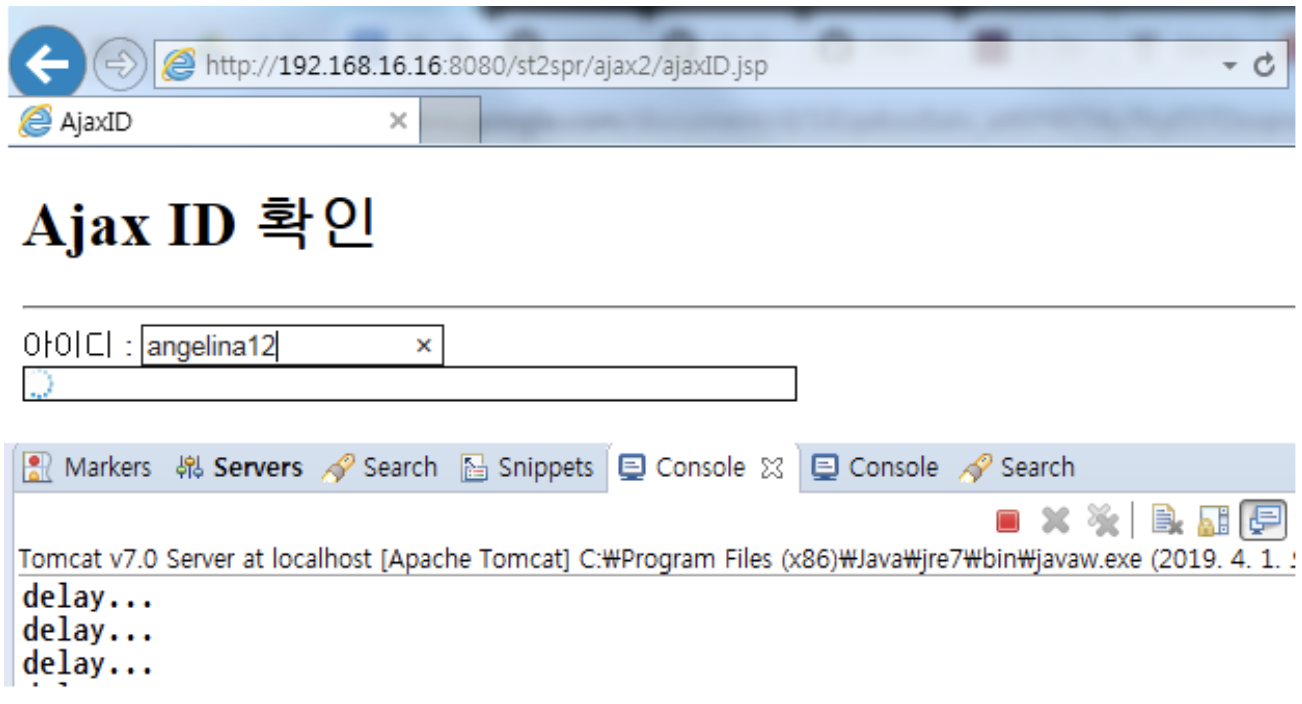
4. Ajax를 활용한 서버 & 클라이언트 시간 체크하기
클라이언트의 시간과 서버의 시간을 ajax로 조회해보고자 한다. 서버의 시간을 넘겨줄 필요는 없지만 데이터를 전송해야 반환값이 오므로 dummy로 보낸다. 이 전송되는 데이터는 클라이언트의 시간이지 서버의 시간이 아니므로 사용하지는 않는다.
① clock.jsp - 요청 페이지
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> <script type="text/javascript"> var XMLHttpRequest; function printClientTime(){ var clientTimeSpan = document.getElementById("clientTimeSpan"); var now = new Date(); var timeStr = now.getFullYear() +"년 "+ (now.getMonth()+1) +"월 " +now.getDate()+"일 " +now.getHours()+"시 " +now.getMinutes()+"분 " +now.getSeconds() + "초 "; clientTimeSpan.innerHTML = timeStr; //1초마다 자기자신을 실행 setTimeout("printClientTime()", 1000); } function requestTime(){ XMLHttpRequest = new ActiveXObject("Msxml2.XMLHTTP"); XMLHttpRequest.open("GET","clock_ok.jsp?dummy="+new Date().getDate(),true); XMLHttpRequest.onreadystatechange = printServerTime; XMLHttpRequest.send(null); //1초마다 자기자신을 실행 setTimeout("requestTime()",1000); } function printServerTime(){ if(XMLHttpRequest.readyState==4){ if(XMLHttpRequest.status==200){ //서버 응답 정상일 때 var serverTimeSpan = document.getElementById("serverTimeSpan"); serverTimeSpan.innerHTML = XMLHttpRequest.responseText; }else{ //200이 아닌경우 alert("오류!"); } }else{ //4이 아닌경우 } } //처음에 호출하는 명렁어 필요 window.onload = function(){ printClientTime(); //client시간 requestTime(); //server시간 } </script> </head> <body> <h1>Clock</h1> <br/><br/> <hr/> 1. 현재 클라이언트 시간은 <span id="clientTimeSpan"></span>입니다. <br/> 2. 현재 서버 시간은 <span id="serverTimeSpan"></span>입니다. <br/> </body> </html> |
② clock_ok.jsp
<%@page import="java.util.Date"%> <%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); //클라이언트 캐쉬 제거 response.setHeader("Pragma", "no-cache"); response.setHeader("Cache-Control", "no-store");//HTTP 1.0버전 response.setHeader("Cache-Control", "no-cache");//HTTP 1.1버전 //세션 삭제 후(로그아웃) 뒤로가기 방지 response.setDateHeader("Expires", 0); %> <%=new Date()%> |
서버PC의 시간을 변동한 뒤 조회하면 각기 다른 시간이 뜸

5. Ajax를 활용한 CSV데이터 읽어오기 - 실시간 뉴스타이틀 구현
Ajax를 사용하다보니 반복해서 사용되는 부분이 존재하여 이걸 메소드로 만들어 사용하고자 한다.
객체생성 var XMLHttpRequest = new ActiveXObject("Msxml2.XMLHTTP");
요청초기화 XMLHttpRequest.open(전송방식,주소,true);
콜백함수 XMLHttpRequest.onreadystatechange = printServerTime;
전송 XMLHttpRequest.send(null);
① httpRequest.js 생성
//XMLHttpRequest 객체 생성 function getXMLHttpRequest(){ if(window.ActiveXObject){ try { return new ActiveXObject("Msxml2.XMLHTTP"); //IE5.0이후 } catch (e) { try { return new ActiveXObject("Microsoft.XMLHTTP"); //IE5.0이전 } catch (e) { return null; } } }else if(window.XMLHttpRequest){ return new XMLHttpRequest(); //non IE }else{ return null; } } //Ajax 요청, 처리작업 var httpRequest = null; function sendRequest(url,params,callback,method){ httpRequest = getXMLHttpRequest(); //method처리 var httpMethod = method?method:"GET"; //method가 있으면 그 내용을 담고, null일 경우 GET으로 셋팅 if(httpMethod!="GET" && httpMethod!="POST"){ httpMethod = "GET"; } //params처리 var httpParams = (params==null||params=="")?null:params; //url처리 var httpUrl = url; if(httpMethod=="GET" && httpParams!=null){ httpUrl += "?" + httpParams; } httpRequest.open(httpMethod,httpUrl,true);//post방식일 경우 httpRequest.setRequestHeader("Content-Type","application/x-www-form-urlencoded"); httpRequest.onreadystatechange = callback; httpRequest.send(httpMethod=="POST"?httpParams:null); } |
② newsTitleCSV.jsp - 요청 페이지
뉴스의 타이틀을 가져올 것. csv 형식으로 (콤마로 구분된 데이터)
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>NewTitle</title> <style type="text/css"> .news{ font-size:10pt; display:block; margin: 0 auto; background: yellow; color:blue; border: 1px dashed black; width: 50% } .newsError{ font-size:10pt; display:block; margin: 0 auto; background: red; color:red; border: 1px dashed black; width: 50% } </style> <script type="text/javascript" src="<%=cp%>/data/js/httpRequest.js"></script> <script type="text/javascript" > function newsTitle(){ //hideNewsDiv();//첫 실행시 안보이도록 sendRequest("newsTitleCSV_ok.jsp", null, displayNewsTitle, "GET");//get방식으로 보내므로 파라미터 null setTimeout("newsTitle()",3000);//3초마다 자기자신 호출 } function displayNewsTitle(){ if(httpRequest.readyState==4){ if(httpRequest.status==200){ var csvStr = httpRequest.responseText;//응답을 텍스트로 받음 var csvArray = csvStr.split("|"); var countStr = csvArray[0]; //alert(countStr);//데이터갯수 if(countStr==0){ alert("News가 없다!"); return; } var csvData = csvArray[1]; //alert(csvData);//데이터 var newTitleArray = csvData.split("*"); //alert(newTitleArray.length+"개");//뉴스제목갯수 var html = ""; html += "<ol>"; for(var i=0;i<newTitleArray.length;i++){ var newsTitle = newTitleArray[i]; html +="<li>"+newsTitle+"</li>"; } html += "</ol>"; //alert(html); var newsDiv = document.getElementById("newsDiv"); newsDiv.innerHTML = html; }else{ var newsDiv = document.getElementById("newsDiv"); newsDiv.innerHTML = httpRequest.status + ":에러발생"; //IE인 경우 newsDiv.className = "newsError"; //IE가 아닌 경우 newsDiv.setAttribute("class","newsError") ; } } } function showNewsDiv(){ //뉴스 보이게 설정 var newsDiv = document.getElementById("newsDiv"); newsDiv.style.display = "block"; } function hideNewsDiv(){ //뉴스 안보이게 설정 var newsDiv = document.getElementById("newsDiv"); newsDiv.style.display = "none"; } window.onload = function(){ newsTitle(); } </script> </head> <body> <h2>헤드라인 뉴스</h2> <hr/> <br/> <!--<div style="display: block; border: 3px solid; width:50%; margin: 0 auto;"> 뉴스보기 </div> <div id="newsDiv" class="news"> </div> </body> </html> |
③ newsTitleCSV_ok.jsp
<%@page import="java.util.Date"%> <%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); //클라이언트 캐쉬 제거 response.setHeader("Pragma", "no-cache"); response.setHeader("Cache-Control", "no-store");//HTTP 1.0버전 response.setHeader("Cache-Control", "no-cache");//HTTP 1.1버전 //세션 삭제 후(로그아웃) 뒤로가기 방지 response.setDateHeader("Expires", 0); %> <%! compile된 데이터를 보면 !가 없으면 Service의 바깥에 변수가 생성된다. (지역변수) 그러므로 !를 넣어서 전역변수로 설정해주어야한다. 웹서버에 컴파일된 서블릿클래스가 생성되는 경로 : D:\java\work\.metadata\.plugins\org.eclipse.wst.server.core\tmp0\work\Catalina\localhost\st2spr\org\apache\jsp\ajax3 String[] newsTitle = { "ㅎㅎ 2000호 특별기획 '핀테크' 성지 룩셈부르크를 가다 ...", "강화된 외감... 대기업마저 '회계포비아'", "반도체株 바닥 다지고 상승할까 ...", "알아두면 유용한 보험계약 관리 노하우", "[신율의 정치 읽기] '민족주의' '이념대립' ...", "[나기자의 Activity] 일렉트로복싱 해보니", "[카드뉴스] 'SUV 게 섰거라' 세단의 반격" }; %> <% //한번에 읽어오는 데이터의 개수가 존재. //내가 어떤 모양으로 출력하겠다는 사용자 정의 //7|2000호 특별기획 '핀테크' 성지 룩셈부르크를 가다 | ...[2019.4.1 오후 4:14:10]* //강화된 외감법… 대기업마저 '회계포비아'[2019.4.1 오후 4:14:10]* out.print(newsTitle.length+"|");//뉴스 갯수 for(int i=0; i<newsTitle.length;i++){ out.print(newsTitle[i]+"["+new Date() +"]");//뉴스시간 if(i!=(newsTitle.length-1)){ out.print("*");//뉴스 제목 끝날때마다 * 찍기. 마지막 뉴스는 찍히지않음 } } //하나의 텍스트로 출력 %> |
실행 화면
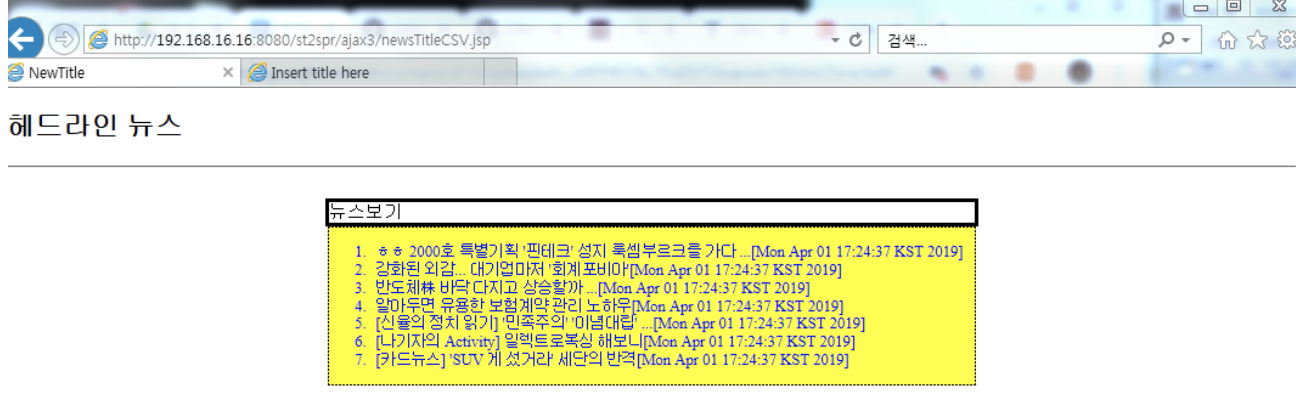
<%! %>로 설정하여 전역변수로 사용된 String[] newsTitle을 확인할 수 있다.
'Dev > JS & Jquery' 카테고리의 다른 글
Ajax - Document Object Model과 XML (0) | 2019.04.03 |
---|---|
Ajax - 검색시 제시어 기능 만들기 (0) | 2019.04.02 |
Ajax - 텍스트 데이터 전송받기 (1) | 2019.03.29 |
JQuery 셋팅 및 예제 (0) | 2019.03.29 |
Ajax란 (0) | 2019.03.29 |