Annotation
스프링을 사용하는 가장 큰 이유 중 하나. 코딩을 단순화 시키는 작업. 객체 생성이 단순해짐
1. Command 클래스 생성
2. JSP페이지 생성 - created.jsp, result.jsp
3. 어노테이션을 사용을 위해 정의 - dispatcher servlet
4. Controller 클래스 생성. 컨트롤러는 클래스이기 때문에 객체를 생성해야 사용이 가능.
어노테이션을 쓸 경우 디스팻처 서블릿에서 작성했던 bean객체를 할 필요가 없어짐
1. Command 클래스 생성
package com.anno; public class TestCommand {//DTO private String userId; private String userName; private String mode; public String getUserId() { return userId; } public void setUserId(String userId) { this.userId = userId; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getMode() { return mode; } public void setMode(String mode) { this.mode = mode; } } |
2-1. JSP페이지 생성 - created.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form action="<%=cp%>/demo/write.action" method="post"> 아이디 : <input type="text" name="userId"/><br/> 이름 : <input type="text" name="userName"/><br/> <input type="submit" value="로그인"/><br/>
</form>
</body>
</html> |
2-2. JSP페이지 생성 - result.jsp
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> ${message } </body> </html> |
3. 어노테이션을 사용하겠다고 정의하는 것이 필요 - dispatcher servlet
어노테이션을 사용할 패키지를 작성해주면 된다. 하지만 대부분 사용할 경우 전체 프로젝트에서 사용함 <context:component-scan base-package="*" scoped-proxy="no"/> <bean class="org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping" p:alwaysUseFullPath="true"> <property name="order" value="1"/> </bean> 가상의 이름이지만 폴더의 이름을 쓰겠다는게 true (만약 서블릿 경로를 포함한 전체 경로를 이용해서 매핑 여부를 판단하도록 설정). 어노테이션을 가장 먼저 실행할 것이므로 order 프로퍼티를 1로 설정 |
4. Controller 클래스 생성
컨트롤러 한 곳에서 매핑정보와 객체 생성 등 모두 정의하기 때문에 직관적이고 오류를 찾기 쉽다.
@Controller("anno.testController") 이 한줄로 인해 스프링이 테스트컨트롤러 클래스를 컨트롤러로 인지하고 <bean name="TestContorller" class="com.anno.TestContorller"/>로 객체 생성한것과 동일하다. 어노테이션으로 객체를 생성할 경우 팀으로 만들어 합칠떄 충돌하는 문제가 발생할 수 있음. 그래서 구분작업을 진행한다. 이름을 지정하는 것이 가능. “패키지명.원하는객체명” @RequestMapping(value="/demo/write.action",method={RequestMethod.GET}) 주소를 입력하게되면 주소를 찾아가서 어느 메소드를 실행해야되는지 작업해줄 것 package com.anno; import javax.servlet.http.HttpServletRequest; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; //컨트롤러 객체생성 @Controller("anno.testController") public class TestController {
//Get방식으로 이주소가 들어오면 write메소드를 찾아가라. 이게 하나의 세트 @RequestMapping(value="/demo/write.action",method={RequestMethod.GET}) public String write() throws Exception{//스프링은 매개변수가 필요할 경우 작성해주면 됨 //스트럿츠2는 메소드안에 변수를 받아오는 코드를 작성해주는 게 차이점. return "anno/created"; //직관적으로 움직임 } //주소가 동일하더라도 메소드방식이 다를경우 write_ok 메소드 실행. //스프링에서는 메소드의 이름이 중요치 않음. 충돌만 나지 않으면 된다 //created.jsp에서 데이터가 전송될때 form태그안에 submit을 통해 항상 post로 전송됨을 기억 @RequestMapping(value="/demo/write.action",method={RequestMethod.POST}) public String write_ok(TestCommand command, HttpServletRequest request) throws Exception{ String message = "이름: "+ command.getUserName(); message += ",아이디: " + command.getUserId() ; //반환값을 보내기 위해 request필요 request.setAttribute("message", message); return "anno/result"; } } |
5. 실행 결과
GET방식과 POST방식을 합친 예시
1.test.jsp 생성
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form action="<%=cp%>/demo/save.action" method="post"> 아이디 : <input type="text" name="userId"/><br/> 이름 : <input type="text" name="userName"/><br/> <input type="hidden" name="mode" value="insert"/> <input type="submit" value="로그인"/><br/> </form> </body> </html> |
2. 컨트롤러에 메소드 추가
//컨트롤러 객체생성 @Controller("anno.testController") public class TestController { //GET방식과 POST방식 둘다 하나의 메소드를 호출 @RequestMapping(value="/demo/save.action",method={RequestMethod.GET,RequestMethod.POST}) public String save(TestCommand command, HttpServletRequest request) throws Exception{ if(command==null||command.getMode()==null||command.getMode().equals("")){ return "anno/test"; } String message = "이름: "+ command.getUserName(); message += ",아이디: " + command.getUserId() ; request.setAttribute("message", message); return "anno/result"; } } |
3. 실행 결과
Command가 아닌 일부 변수만 전송 가능
1. View 생성 (입력 JSP 페이지)
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <form action="<%=cp%>/demo/demo.action" method="post"> 아이디 : <input type="text" name="userId"/><br/> 이름 : <input type="text" name="userName"/><br/> <input type="hidden" name="mode" value="insert"/> <input type="submit" value="로그인"/><br/> </form> </body> </html> |
2. Controller 생성
//컨트롤러 객체생성 @Controller("anno.testController") public class TestController { //Command 전체가 아니라 일부 파라미터만 사용하는 것도 가능하다 @RequestMapping(value="/demo/demo.action",method={RequestMethod.GET,RequestMethod.POST}) public String save(String userId, String userName, String mode, HttpServletRequest request, HttpServletResponse response) throws Exception{ if(mode==null||mode.equals("")) return "anno/demo";
String message = "이름: "+ userName; message += ",아이디: " + userId ; request.setAttribute("message", message); return "anno/result"; } } |
3. 실행 페이지
@RequestMapping(value="/main.action", method={RequestMethod.GET}) 요청uri와 RequestMethod를 한꺼번에 혹은 따로 기재할 수 있다.
1. jsp페이지 생성
<%@ page contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <% request.setCharacterEncoding("UTF-8"); String cp = request.getContextPath(); %> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <h1>메인화면이에요</h1> <a href="<%=cp %>/demo/write.action">created</a> <a href="<%=cp %>/demo/save.action">save</a> <a href="<%=cp %>/demo/demo.action">demo</a> </body> </html> |
2. 컨트롤러 생성
RequestMapping을 한번에 작성할 수 있지만 원래는 나눠 작성.
ckage com.anno; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller @RequestMapping(value="/main.action") public class MainController {
@RequestMapping(method=RequestMethod.GET) public String method(){ return "/main"; } } |
3. 실행 페이지
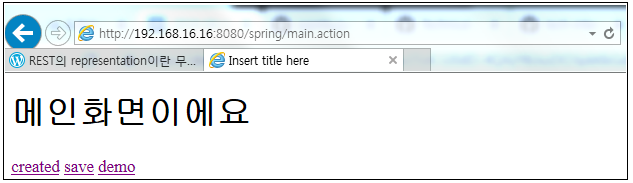
'Dev > Spring' 카테고리의 다른 글
Spring2.5 - 파일게시판(업로드/다운로드/삭제) (0) | 2019.04.08 |
---|---|
Spring2.5 - 게시판 만들기 (0) | 2019.04.08 |
Spring2.5 MVC - MultiActionController (0) | 2019.04.05 |
Spring2.5 MVC - AbstractWizardFormController (0) | 2019.04.05 |
Spring2.5 MVC - Exception & SimpleFormController (0) | 2019.04.05 |